Home » Questions » Computers | [ Ask a new question ] |
Traverse path up and check/set permissions in shell script - how?
Traverse path up and check/set permissions in shell script - how? | |
Asked by: Guest | Views: 319 |
Total answers/comments: 2 | |
| |
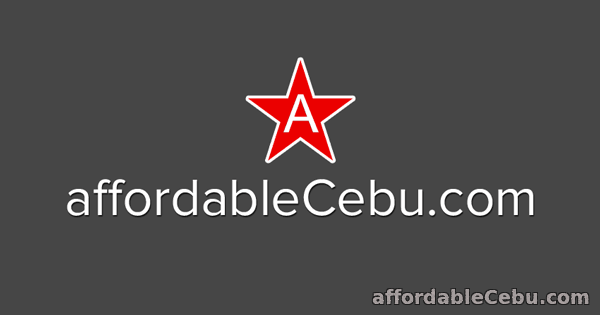
Home » Questions » Computers | [ Ask a new question ] |
Traverse path up and check/set permissions in shell script - how? | |
Asked by: Guest | Views: 319 |
Total answers/comments: 2 | |
| |