Home » Questions » Computers | [ Ask a new question ] |
datetime - Calculate relative time in C# -
"Given a specific DateTime value, how do I display relative time, like: | |
Asked by: Guest | Views: 317 |
Total answers/comments: 3 | |
| |
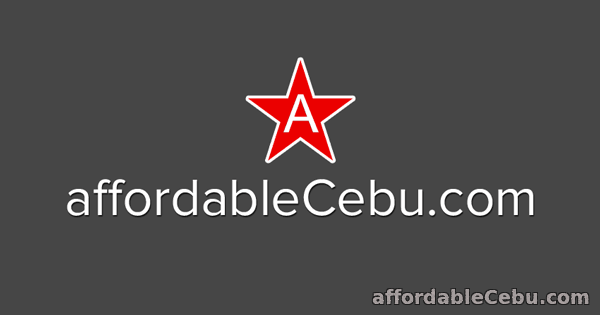
Home » Questions » Computers | [ Ask a new question ] |
"Given a specific DateTime value, how do I display relative time, like: | |
Asked by: Guest | Views: 317 |
Total answers/comments: 3 | |
| |