Home » Questions » Computers | [ Ask a new question ] |
How can I get the comment of the current authorized_keys ssh key?
How can I get the comment of the current authorized_keys ssh key? | |
Asked by: Guest | Views: 258 |
Total answers/comments: 2 | |||
| |||
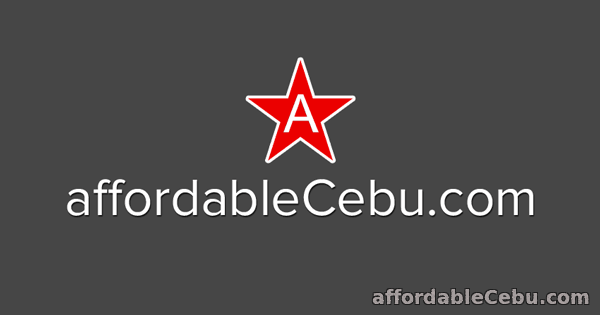
Home » Questions » Computers | [ Ask a new question ] |
How can I get the comment of the current authorized_keys ssh key? | |
Asked by: Guest | Views: 258 |
Total answers/comments: 2 | |||
| |||