Home » Questions » Computers | [ Ask a new question ] |
Making BASH script `for` handle filenames with spaces (or workaround)
Making BASH script `for` handle filenames with spaces (or workaround) | |
Asked by: Guest | Views: 412 |
Total answers/comments: 5 | |
| |
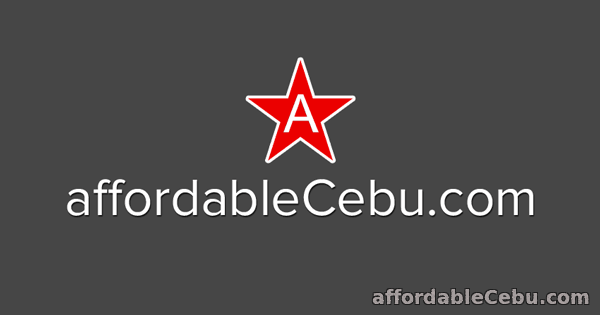