Home » Questions » Computers | [ Ask a new question ] |
GNU BC: "modulo" % with scale other than 0
GNU BC: "modulo" % with scale other than 0 | |
Asked by: Guest | Views: 207 |
Total answers/comments: 2 | |
| |
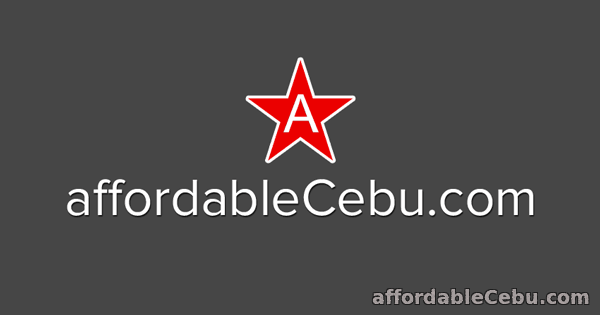
Home » Questions » Computers | [ Ask a new question ] |
GNU BC: "modulo" % with scale other than 0 | |
Asked by: Guest | Views: 207 |
Total answers/comments: 2 | |
| |